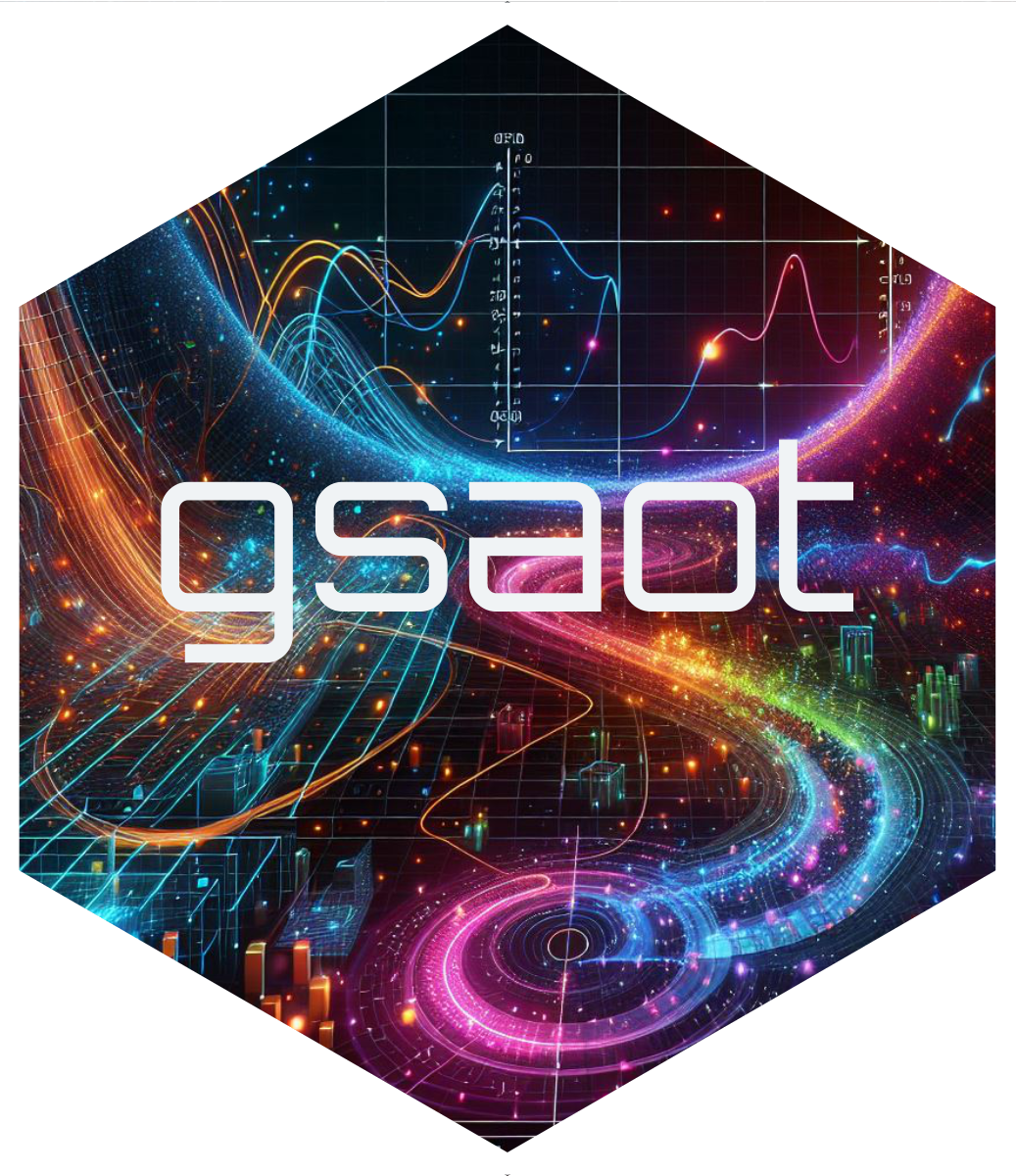
Calculate Optimal Transport sensitivity indices for multivariate y
Source:R/ot_indices.R
ot_indices.Rd
ot_indices
calculates sensitivity indices using Optimal
Transport (OT) for a multivariate output sample y
with respect to input
data x
. Sensitivity indices measure the influence of inputs on outputs,
with values ranging between 0 and 1.
Usage
ot_indices(
x,
y,
M,
cost = "L2",
discrete_out = FALSE,
solver = "sinkhorn",
solver_optns = NULL,
scaling = TRUE,
boot = FALSE,
stratified_boot = FALSE,
R = NULL,
parallel = "no",
ncpus = 1,
conf = 0.95,
type = "norm"
)
Arguments
- x
A matrix or data.frame containing the input(s) values. The values can be numeric, factors, or strings. The type of data changes the partitioning. If the values are continuous (double), the function partitions the data into
M
sets. If the values are discrete (integers, strings, factors), the number of partitioning sets is data-driven.- y
A matrix containing the output values. Each column represents a different output variable, and each row represents a different observation. Only numeric values are allowed.
- M
A scalar representing the number of partitions for continuous inputs.
- cost
(default
"L2"
) A string or function defining the cost function of the Optimal Transport problem. It should be "L2" or a function taking as input y and returning a cost matrix. Ifcost="L2"
,ot_indices
uses the squared Euclidean metric.- discrete_out
(default
FALSE
) Logical, by default the output sample iny
are equally weighted. Ifdiscrete_out=TRUE
, the function tries to create an histogram of the realizations and to use the histogram as weights. It works if the output is discrete or mixed and the number of realizations is large. The advantage of this option is to reduce the dimension of the cost matrix.- solver
Solver for the Optimal Transport problem. Currently supported options are:
"sinkhorn"
(default), the Sinkhorn's solver cuturi2013sinkhorngsaot."sinkhorn_log"
, the Sinkhorn's solver in log scale peyre2019computationalgsaot."transport"
, a solver of the non regularized OT problem usingtransport::transport()
.
- solver_optns
(optional) A list containing the options for the Optimal Transport solver. See details for allowed options and default ones.
- scaling
(default
TRUE
) Logical that sets whether or not to scale the cost matrix.- boot
(default
FALSE
) Logical that sets whether or not to perform bootstrapping of the OT indices.- stratified_boot
(default
FALSE
) Logical that sets the type of resampling performed. Withstratified_boot=FALSE
, the function resamples the dataset and then creates the partitions. Otherwise, first, it creates the partitions and then it performs stratified bootstrapping with strata being the partitions.- R
(default
NULL
) Positive integer, number of bootstrap replicas.- parallel
(default
"no"
) The type of parallel operation to be used (if any). If missing, the default is taken from the optionboot.parallel
(and if that is not set,"no"
). Only considered ifboot = TRUE
. For more information, check theboot::boot()
function.- ncpus
(default
1
) Positive integer: number of processes to be used in parallel operation: typically one would chose this to the number of available CPUs. Check thencpus
option in theboot::boot()
function of the boot package.- conf
(default
0.95
) Number between0
and1
representing the confidence level. Only considered ifboot = TRUE
.- type
(default
"norm"
) Method to compute the confidence interval. Only considered ifboot = TRUE
. For more information, check thetype
option ofboot::boot.ci()
.
Value
A gsaot_indices
object containing:
method
: a string that identifies the type of indices computed.indices
: a names array containing the sensitivity indices between 0 and 1 for each column in x, indicating the influence of each input variable on the output variables.bound
: a double representing the upper bound of the separation measure or an array representing the mean of the separation for each input according to the bootstrap replicas.x
,y
: input and output data provided as arguments of the function.inner_statistic
: a list of matrices containing the values of the inner statistics for the partitions defined bypartitions
. Ifmethod = wasserstein-bures
, each matrix has three rows containing the Wasserstein-Bures indices, the Advective, and the Diffusive components.partitions
: a matrix containing the partitions built to calculate the sensitivity indices. Each column contains the partition associated to the same column inx
. Ifboot = TRUE
, the object contains also:indices_ci
: adata.frame
with first column the input, second and third columns the lower and upper bound of the confidence interval.inner_statistic_ci
: a list of matrices. Each element of the list contains the lower and upper confidence bounds for the partition defined by the row.bound_ci
: a list containing the lower and upper bounds of the confidence intervals of the separation measure bound.type
,conf
: type of confidence interval and confidence level, provided as arguments.
Details
Solvers
OT is a widely studied topic in Operational Research and Calculus. The
reference for the OT solvers in this package is
peyre2019computational;textualgsaot. The default solver is
"sinkhorn"
, the Sinkhorn's solver introduced in
cuturi2013sinkhorn;textualgsaot. It solves the
entropic-regularized version of the OT problem. The "sinkhorn_log"
solves
the same OT problem but in log scale. It is more stable for low values of
the regularization parameter but slower to converge. The option
"transport"
is used to choose a solver for the non-regularized OT
problem. Under the hood, the function calls transport::transport()
from
package transport
. This option does not define the solver per se, but the
solver should be defined with the argument solver_optns
. See the next
section for more information.
Solver options
The argument solver_optns
should be empty (for default options) or a list
with all or some of the required solver parameters. All the parameters not
included in the list will be set to default values. The solvers
"sinkhorn"
and "sinkhorn_log"
have the same options:
numIterations
(default1e3
): a positive integer defining the maximum number of Sinkhorn's iterations allowed. If the solver does not converge in the number of iterations set, the solver will throw an error.epsilon
(default0.01
): a positive real number defining the regularization coefficient. If the value is too low, the solver may returnNA
.maxErr
(default1e-9
): a positive real number defining the approximation error threshold between the marginal histogram of the partition and the one computed by the solver. The solver may fail to converge innumIterations
if this value is too low.
The solver "transport"
has the parameters:
method
(default"networkflow
): string defining the solver of the OT problem.control
: a named list of parameters for the chosen method or the result of a call totransport::trcontrol()
.threads
(default1
): an Integer specifying the number of threads used in parallel computing.
For details regarding this solver, check the transport::transport()
help
page.
Examples
N <- 1000
mx <- c(1, 1, 1)
Sigmax <- matrix(data = c(1, 0.5, 0.5, 0.5, 1, 0.5, 0.5, 0.5, 1), nrow = 3)
x1 <- rnorm(N)
x2 <- rnorm(N)
x3 <- rnorm(N)
x <- cbind(x1, x2, x3)
x <- mx + x %*% chol(Sigmax)
A <- matrix(data = c(4, -2, 1, 2, 5, -1), nrow = 2, byrow = TRUE)
y <- t(A %*% t(x))
x <- data.frame(x)
M <- 25
# Calculate sensitivity indices
sensitivity_indices <- ot_indices(x, y, M)
sensitivity_indices
#> Method: sinkhorn
#>
#> Solver Options:
#> $numIterations
#> [1] 1000
#>
#> $epsilon
#> [1] 0.01
#>
#> $maxErr
#> [1] 1e-09
#>
#>
#> Indices:
#> X1 X2 X3
#> 0.5982222 0.6120543 0.2761801
#>
#> Upper bound: 95.02202